Element library
Here you will find examples of most used CSS elements and how to implement them.
Background and color
Add border
Height
Progress color
Progress height
Steps
Active
Inactive
Import
Adding fonts
Size
Font color
Headings
Forms
Links
Flat text fields
Bevel text fields
Corners
Shadows
Buttons
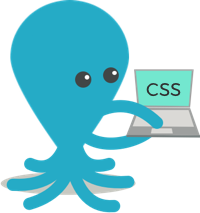
Steve the Octopus knows his CSS.
Background
Color
Change the color of your text
body {
background: #ffffff
}
Image
You should also add an background color. If the image is inaccessible, it will revert to the defined color.
Size
The suggested size of the background image is 1920 x 1080px.
body {
background: #ffffff url("path to your image");
}
Static image
Static background image
Background positions
Positions of the background image define where you want the image to anchor to on the page.
body {
background: url("path to your image") bottom center;
background-size: cover;
background-attachment: fixed;
}
Navigation
Background and color
Change navigation bar's background and text color
.navbar-default {
background: #ffffff;
color: #000000;
}
.container {
background: none;
}
Add border
Add a border with color to the bottom of the navigation bar
.navbar-default {
border-bottom: 2px solid #000000;
}
Height
Change height of navigation bar, changing values as necessary
.navbar-nav > li > a {
margin: 20px 0;
}
Progress color
Change progress bar's colors
.progress and .progress-bar
.progress is the whole bar itself and .progress-bar displays progress that has been done.
.progress {
background: #ffffff;
}
.progress-bar {
background: #000000;
}
Progress height
Change the height of progress bar
.progress {
height: 6px;
}
.progress-bar {
height: 6px;
}
Steps
Change color of the text of steps that have been completed in navigation bar and the color of it while hovering
.navbar-default .navbar-nav > li > a {
color: #000000;
}
.navbar-default .navbar-nav > li > a:hover {
color: #FF0000;
}
Active
Change style of the text in navigation bar that is currently active
.navbar-default .navbar-nav>.active>a, .navbar-default .navbar-nav>.active>a:hover, .navbar-default .navbar-nav>.active>a:focus {
color: #000000;
}
Inactive
Change style of inactive
.navbar-default .navbar-nav>.disabled>a, .navbar-default .navbar-nav>.disabled>a:hover, .navbar-default .navbar-nav>.disabled>a:focus {
color: #000000;
opacity: 0.6;
}
Fonts
Fallback
It's always good to have 'sans serif' defined as the fallback font. It will use any font on the users computer that is sans serif if no other fonts can be loaded.
Import
Import font file — eg. from Google fonts — and use it as the default font
This needs to go in the beginning of the file
<style type="text/css">
@import url('https://fonts.googleapis.com/css?family=Open+Sans');
body {
font-family: 'Open Sans', sans-serif;
}
</style>
Adding font
Change font face from your own font file
This needs to go to the beginning of the file
You can add multiple sources to one font file for better combatibility.
<style type="text/css">
@font-face {
src: url("Path to your font file");
font-family: 'Name of your font', sans-serif;
}
body {
font-family: 'Name of your font';
}
</style>
Size
Change the size of the font of body text
body {
font-family: 'Name of the font', sans-serif;
font-size: 16px;
}
Font color
Change the color of the font
body {
color: #000000;
}
Headings
Change headings' color and size
h1, h2, h3, h4, h5, h6 {
color: #F00;
font-family: 'Name of your font', sans-serif;
}
h1 {
font-size: 22px;
}
h2 {
font-size: 20px;
}
h3 {
font-size: 18px;
}
h4 {
font-size: 16px;
}
h5 {
font-size: 15px;
}
h6 {
font-size: 14px;
}
Forms
Change font for forms
.form-control {
font-family: 'Name of your font', sans-serif;
}
Content area
Background opacity
Change content area's opacity
#letter-container-responsive contains all the content in the middle, including banners.
#common-content is inside #letter-container-responsive and contains all content elements.
#letter-container-responsive {
background: rgba(255,255,255,0.5);
}
#common-content {
background: rgba(255,255,255,0);
}
Background
Change content area's background color
#letter-container-responsive {
background: #ffffff;
}
#common-content {
background: #ffffff;
}
Interactive
Links
Change the style of links
a {
color: #0000FF;
cursor: pointer;
}
a:hover, a:focus {
color: #0000FF;
text-decoration: underline;
}
Flat text field
Change input fields to flat style
.form-control {
box-shadow: none;
border-color: #cccccc;
}
Bevel text field
Change input fields to bevel style
.form-control {
box-shadow: inset 0 0 1px rgb(128,128,128);
border-color: #cccccc;
}
.form-control:focus {
box-shadow: inset 0 0 2px rgb(128,128,128);
border-color: #cccccc;
}
Buttons
Change buttons' backgrounds, borders and text colors
Use relevant selectors for right buttons. Try them out!
.btn-success, .btn-primary {
background: #000000;
color: #ffffff;
border: 1px solid #000000;
}
.btn-success:hover, .btn-success:focus,.btn-success:active, .btn-primary:hover, .btn-primary:focus, .btn-primary:active {
background: #ffffff;
color: #000000;
border: 1px solid #ffffff;
}
.btn-default {
background: #000000;
color: #ffffff;
border: 1px solid #000000;
}
.btn-default:hover, .btn-default:active, .btn-default:focus {
background: #ffffff;
color: #000000;
border: 1px solid #ffffff;
}
#thanks-page-button-0, #thanks-page-button-1, #thanks-page-button-2, #thanks-page-button-3, #thanks-page-button-4, #thanks-page-button-5, #thanks-page-button-6 {
background: #ffffff;
color: #000000;
border: 1px solid #ffffff;
}
#thanks-page-button-0:hover, #thanks-page-button-1:hover, #thanks-page-button-2:hover, #thanks-page-button-3:hover, #thanks-page-button-4:hover, #thanks-page-button-5:hover, #thanks-page-button-6:hover {
background: #ffffff;
color: #000000;
border: 1px solid #ffffff;
}
Corners
Change buttons' corners roundness
.btn {
border-radius: 20px;
}
Shadows
Give a shadow to the buttons
.btn {
box-shadow: 0 2px 2px #000000;
}
Updated over 4 years ago